BB-8
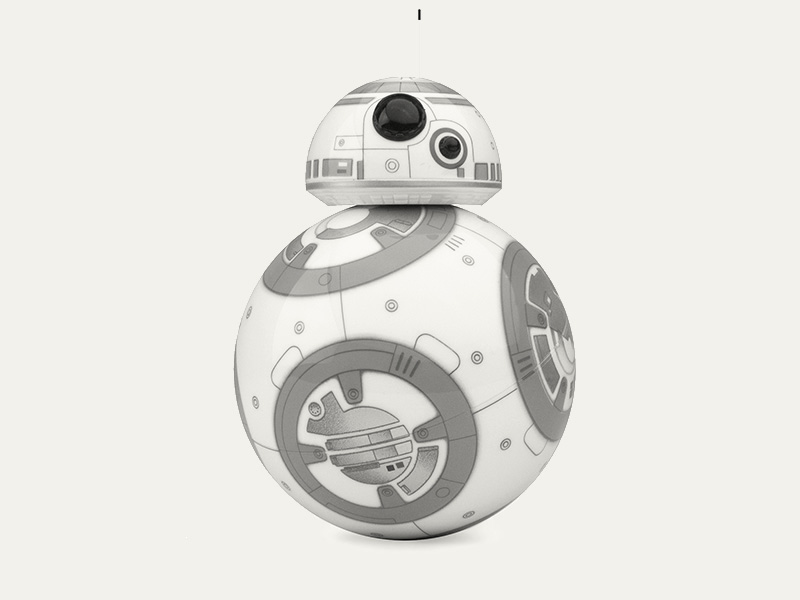
Allows user to send commands to Sphero BB-8. API Reference
How To Connect
The Sphero BB-8 is a Bluetooth LE device. You need to know the BLE ID of the BB-8 you want to connect to. The Gobot BLE client adaptor also lets you connect by friendly name, aka "BB-1247".
import ( "gobot.io/x/gobot/platforms/ble" "gobot.io/x/gobot/platforms/sphero/bb8" ) bleAdaptor := ble.NewClientAdaptor(os.Args[1]) bb8 := bb8.NewDriver(bleAdaptor) ...
How To Use
package main import ( "os" "time" "gobot.io/x/gobot" "gobot.io/x/gobot/platforms/ble" "gobot.io/x/gobot/platforms/sphero/bb8" ) func main() { bleAdaptor := ble.NewClientAdaptor(os.Args[1]) bb8 := bb8.NewDriver(bleAdaptor) work := func() { gobot.Every(1*time.Second, func() { r := uint8(gobot.Rand(255)) g := uint8(gobot.Rand(255)) b := uint8(gobot.Rand(255)) bb8.SetRGB(r, g, b) }) } robot := gobot.NewRobot("bb", []gobot.Connection{bleAdaptor}, []gobot.Device{bb8}, work, ) robot.Start() }