Digispark
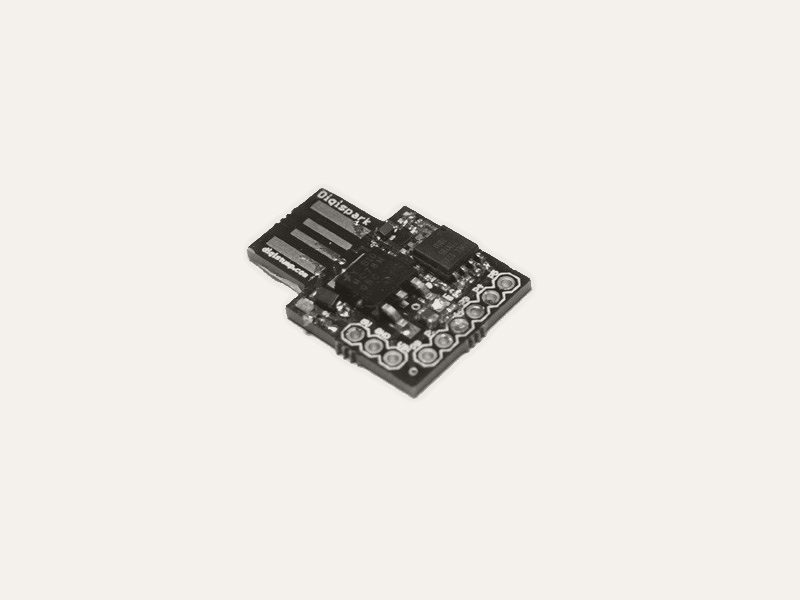
The Digispark is an Attiny85 based microcontroller development board similar to the Arduino line, only cheaper, smaller, and a bit less powerful. With a whole host of shields to extend its functionality and the ability to use the familiar Arduino IDE the Digispark is a great way to jump into electronics, or perfect for when an Arduino is too big or too much.
This package provides the Gobot adaptor for the Digispark ATTiny-based USB development board with the Little Wire protocol firmware installed.
API ReferenceHow to Install
This package requires libusb
.
OSX
To install libusb
on OSX using Homebrew:
$ brew install libusb && brew install libusb-compat
Ubuntu
To install libusb on linux:
$ sudo apt-get install libusb-dev
Now you can install the package with
go get -d -u gobot.io/x/gobot/...
How to Use
package main import ( "time" "gobot.io/x/gobot" "gobot.io/x/gobot/drivers/gpio" "gobot.io/x/gobot/platforms/digispark" ) func main() { digisparkAdaptor := digispark.NewAdaptor() led := gpio.NewLedDriver(digisparkAdaptor, "0") work := func() { gobot.Every(1*time.Second, func() { led.Toggle() }) } robot := gobot.NewRobot("blinkBot", []gobot.Connection{digisparkAdaptor}, []gobot.Device{led}, work, ) robot.Start() }
How to Connect
If your Digispark already has the Little Wire protocol firmware installed, you can connect right away with Gobot.
Otherwise, you must first flash your Digispark with the Little Wire firmware.
The easiest way to flash your Digispark is to use Gort https://gort.io.
Download and install Gort, and then use the following commands:
Then, install the needed Digispark firmware.
gort digispark install
OSX
Important: 2012 MBP The USB ports on the 2012 MBPs (Retina and non) cause issues due to their USB3 controllers, currently the best work around is to use a cheap USB hub (non USB3).
Plug the Digispark into your computer via the USB port and run:
gort digispark upload littlewire
Ubuntu
Ubuntu requires an extra one-time step to set up the Digispark for communication with Gobot. Run the following command:
gort digispark set-udev-rules
You might need to enter your administrative password. This steps adds a udev rule to allow access to the Digispark device.
Once this is done, you can upload Little Wire to your Digispark:
gort digispark upload littlewire
Windows
We need instructions here, because it supposedly works.
Manual instructions
For manual instructions on how to install Little Wire on a Digispark check out http://digistump.com/board/index.php/topic,160.0.html
Thanks to @bluebie for these instructions! (https://github.com/Bluebie/micronucleus-t85/wiki/Ubuntu-Linux)
Now plug the Digispark into your computer via the USB port.
Drivers
Thanks to Gobot and go it is possible to interact with several I/O hardware devices and interfaces connected to the Digispark using a set of already defined and easy to use drivers, all of this thanks to the Gobot architecture, in conjunction with Gobot Digispark adaptor (using the littlewire communication protocol), which makes it even possible to swap the entire platform for something else like Arduino, and keep using the same code you just wrote for Digispark, just by swapping a couple of lines in your program.
Available drivers for the Digispark platform are listed below, the drivers contain detailed documentation and examples of what kind physical computing (using Gobot and Go) you could be doing: